Project Members: 
Aylin Selcukoglu
Jess Kline
kartikeya
n8agrin
Travis Pinnick
Description
It's a box! But it's no ordinary box - it's a drum box.
It's collaborative, like a drum circle, portable, and customizable (i.e. you can upload any beat that you want to map to a drum pad and change it around at will).
Components
A large purple tub (not quite a box - but box sounded better than tub), 4 red leds, 4 fsrs, 4 cardboard circles, bubble wrap, breadboard, resistors, and a lot of wire
Arduino Code
/*
* interface to detect when FSR's have been hit
* in response make LEDs blink and tell Processing that the FSR was pressed
*/
// analog pin settings
int fsrPin1 = 5; // FSRs connected to analog pins 5 - 2
int fsrPin2 = 4;
int fsrPin3 = 3;
int fsrPin4 = 2;
// digital pin settings
int ledPin1 = 11; // LEDs connected to digital pins 13 - 10
int ledPin2 = 10;
int ledPin3 = 9;
int ledPin4 = 8;
// values
int val1 = 0; // variables to store input from FSRs
int val2 = 0;
int val3 = 0;
int val4 = 0;
void setup() {
pinMode(ledPin1, OUTPUT); // set digital pins as output
pinMode(ledPin2, OUTPUT);
pinMode(ledPin3, OUTPUT);
pinMode(ledPin4, OUTPUT);
//TEST
/*digitalWrite(ledPin1, HIGH);
digitalWrite(ledPin2, HIGH);
digitalWrite(ledPin3, HIGH);
digitalWrite(ledPin4, HIGH);*/
Serial.begin(9600); // open serial communication
//Serial.print("go"); // tell Processing Arduino is ready
}
void loop() {
val1 = analogRead(fsrPin1); // read the input pins (FSR's)
val2 = analogRead(fsrPin2);
val3 = analogRead(fsrPin3);
val4 = analogRead(fsrPin4);
//if val above threshold
if(val1 >= 100) {
Serial.println("a"); // FSR A went off, send to Processing
//Serial.println(val1); // send value to Processing
digitalWrite(ledPin1, HIGH); // turn LED on
delay(100);
digitalWrite(ledPin1, LOW); // turn LED off
}
//if val above threshold
if(val2 >= 100) {
Serial.println("b"); // FSR B went off, send to Processing
//Serial.println(val2); // send value to Processing
digitalWrite(ledPin2, HIGH); // turn LED on
delay(100);
digitalWrite(ledPin2, LOW); // turn LED off
}
//if val above threshold
if(val3 >= 100) {
Serial.println("c"); // FSR C went off, send to Processing
//Serial.println(val3); // send value to Processing
digitalWrite(ledPin3, HIGH); // turn LED on
delay(100);
digitalWrite(ledPin3, LOW); // turn LED off
}
//if val above threshold
if(val4 >= 100) {
Serial.println("d"); // FSR D went off, send to Processing
//Serial.println(val4); // send value to Processing
digitalWrite(ledPin4, HIGH); // turn LED on
delay(100);
digitalWrite(ledPin4, LOW); // turn LED off
}
}
Processing Code
// Analysis
// by Krister Olsson <http://www.tree-axis.com>
// extended a tad bit by n8agrin
// Plays an MP3 while displaying an oscilloscope
// and performing real-time spectrum analysis.
// Click to start/stop
// Created 1 May 2005
// Updated 18 May 2006
// Updated for TUI lab, Nov 8th, 2007
import krister.Ess.*;
import processing.serial.*;
// Setup a port
Serial port;
String portname = "/dev/tty.usbserial-A4001nvG";
// Establish output channels and buffers
AudioChannel[] channels = new AudioChannel[4];
int[] bufferSizes = new int[4];
int[] bufferDurations = new int[4];
FFT[] FFTs = new FFT[4];
// bground for the visualization
int bg = 1;
void setup() {
// Create the serial connection
port = new Serial(this, portname, 9600);
// Size of the vis window
size(1024,200);
// start up Ess
Ess.start(this);
// Setup the drum hits
channels[0] = new AudioChannel("drum1.wav");
channels[1] = new AudioChannel("drum2.wav");
channels[2] = new AudioChannel("drum3.wav");
channels[3] = new AudioChannel("drum4.wav");
for (int i=0; i<channels.length; i++){
bufferSizes[i] = channels[i].buffer.length;
bufferDurations[i] = channels[i].ms(bufferSizes[i]);
FFTs[i] = new FFT(512);
}
// set the frame rate for the vis
frameRate(30);
noSmooth(); // no harsh, either, but hey what the heck
}
void draw() {
if (bg < 254) {
bg++;
}
else {
bg = 1;
}
background(bg,110,255);
drawSpectrum();
drawSamples();
}
void drawSpectrum() {
noStroke();
for (int i=0; i < channels.length; i++) {
FFTs[i].getSpectrum(channels[i]);
}
}
void drawSamples() {
stroke(255);
for (int j=0; j < channels.length; j++) {
// interpolate between 0 and writeSamplesSize over writeUpdateTime
int interp=(int)max(0,(((millis()-channels[j].bufferStartTime)/(float)bufferDurations[j])*bufferSizes[j]));
for (int i=0;i<1024;i++) {
float left=100;
float right=100;
if (i+interp+1<channels[j].buffer2.length) {
left-=channels[j].buffer2[i+interp]*75.0;
right-=channels[j].buffer2[i+1+interp]*75.0;
}
line(i,left,i+1,right);
}
}
}
void serialEvent(Serial p) {
playSample((char) p.read());
}
void keyPressed() {
playSample(key);
}
void playSample(char letter) {
switch(letter) {
case 'a':
channels[0].play();
break;
case 'b':
channels[1].play();
break;
case 'c':
channels[2].play();
break;
case 'd':
channels[3].play();
break;
default:
for(int i=0; i<channels.length; i++) {
if (channels[i].state == Ess.PLAYING) {
channels[i].stop();
}
}
break;
}
}
// clean up Ess before exiting
public void stop() {
Ess.stop();
super.stop();
}
Pictures
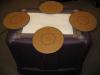
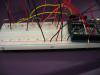