Wind Speed Fan
Description
This project reads data from Yahoo's rss weather feed in xml format. Processing receives the xml data (for a zip code that can be specified in the program) and identifies the current speed of the wind at the specified location. Processing then writes a value to the serial port depending on the wind speed. Arduino receives the serial input and accordingly writes a value to the connected DC motor, which has a fan attached to it. So, in effect, the speed of the fan reflects the actual speed of the wind at the specified location.
Materials
Arduino board, DC motor, fan, battery pack, transistor, diode
Processing Code
/* This program reads in xml data from yahoo's rss weather feed.
* The zip code for the location to use can be entered in the "zip" string field.
* The program processes the xml data and locates the wind speed at the specfied location.
* The program then prints out the current wind speed.
* Depending on the wind speed, a certain value is written to the serial port.
*
* Written by Jon Breitbart with help from the code for project at:
* http://www.doryexmachina.com/projects/arboroptics/
*/
import processing.xml.*;
import processing.serial.*;
XMLElement xml;
int wind = 0;
String zip = "94709";
String portname = "COM4"; // or "COM5"
Serial myPort;
void setup() {
myPort = new Serial(this, portname, 9600);
size(200, 200);
xml = new XMLElement(this, "http://xml.weather.yahoo.com/forecastrss?p=" + zip);
String string = xml.toString();
String name = xml.getName();
XMLElement channel = xml.getChild(0);
int numSites = channel.getChildCount();
XMLElement windspeed = channel.getChild(8);
String speed = windspeed.getStringAttribute("speed");
wind = int (speed);
println("Wind = " + wind + " mph");
}
void draw(){
if (wind < 1){
myPort.write('1');
}
if (wind >= 1 && wind < 3){
myPort.write('2');
}
if (wind >= 3 && wind < 5){
myPort.write('3');
}
if (wind >=5 && wind < 10){
myPort.write('4');
}
if (wind >=10 && wind < 20){
myPort.write('5');
}
if (wind >=20 && wind < 30){
myPort.write('6');
}
if (wind >=30 && wind < 50){
myPort.write('7');
}
if (wind >=50){
myPort.write('8');
}
}
Arduino Code
/*
* modified version of AnalogInput
* by DojoDave <http://www.0j0.org>
* http://www.arduino.cc/en/Tutorial/AnalogInput
* Modified again by dave and then modified by Jon Breitbart
*
* This program reads input from the serial port (from Processing).
* It then writes a value to a motor (which has a fan attached) depending on serial input.
*
* By Jon Breitbart with help from the code for project at:
* http://www.doryexmachina.com/projects/arboroptics/
*/
int motorPin = 9; // select the pin for the Motor
int val = 0; // variable to store the value coming from the serial port
void setup() {
Serial.begin(9600);
}
void loop() {
val = Serial.read(); // read the value from the serial port
if (val == '1'){
analogWrite(motorPin, 0); // write a value to the motor
}
if (val == '2'){
analogWrite(motorPin, 25);
}
if (val == '3'){
analogWrite(motorPin, 50);
}
if (val == '4'){
analogWrite(motorPin, 75);
}
if (val == '5'){
analogWrite(motorPin, 100);
}
if (val == '6'){
analogWrite(motorPin, 130);
}
if (val == '7'){
analogWrite(motorPin, 190);
}
if (val == '8'){
analogWrite(motorPin, 240);
}
}
Picture of Fan
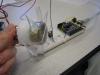
Comments
Comments from TAs
Nice work connecting RSS, Processing, and Arduino. I like the idea and appreciate the work that you put into getting it to work. Good job! It would be interesting to see what other RSS feeds could make good inputs, given the set of outputs that we've worked with... Maybe an RSS-based musical instrument?