Description
I used two potentiometers to control fading and blinking of 3 LEDs.
- Fading: the program cross-fades from red to green, green to blue, and blue to red.
- Blinking: depends on the potentiometer.
Hardware Components Used
3 LED
3 Resistors
2 Potentiometers
Arduino Board
Breadboard
Wires
Arduino Code
/*
* Code for making one potentiometer controls 3 LEDs, red, grn and blu,
* and the other potentiometer controls the time gap between two LEDs.
* The program cross-fades from red to grn, grn to blu, and blu to red.
* You can try 100% smooth fading but also blinking-like fading.
* Modified the code from http://www.arduino.cc/en/Tutorial/LEDCross-fadesWithPotentiometer by Clay Shirky <clay.shirky@nyu.edu>
*
* 09/19/07 Tangible User Interfaces
* Lab 3. Eun Kyoung Choe
*/
// INPUT: Potentiometer should be connected to 5V and GND
int potPin1 = 1; // Potentiometer output connected to analog pin 1, controls fading
int potVal1 = 0; // Variable to store the input from the potentiometer 1
int potPin2 = 2; // Potentiometer output connected to analog pin 2, controls time gap
int potVal2 = 0;
// OUTPUT: Use digital pins 9-11, the Pulse-width Modulation (PWM) pins
// LED's cathodes should be connected to digital GND
int redPin = 9; // Red LED, connected to digital pin 9
int grnPin = 10; // Green LED, connected to digital pin 10
int bluPin = 11; // Blue LED, connected to digital pin 11
// Program variables
int redVal = 0; // Variables to store the values to send to the pins
int grnVal = 0;
int bluVal = 0;
void setup()
{
pinMode(redPin, OUTPUT); // sets the pins as output
pinMode(grnPin, OUTPUT);
pinMode(bluPin, OUTPUT);
}
// Main program
void loop()
{
potVal1 = analogRead(potPin1); // read the potentiometer value at the input pin
potVal2 = analogRead(potPin2) / 5; // reasonable time gap (0~~200)
if (potVal1 < 341) // Lowest third of the potentiometer's range (0-340)
{
potVal1 = (potVal1 * 3) / 4; // Normalize to 0-255
redVal = 255 - potVal1; // Red from full to off
delay(potVal2); // Stop the program for some time
grnVal = potVal1; // Green from off to full
delay(potVal2); // Stop the program for some time
bluVal = 0; // Blue off
}
else if (potVal1 < 682) // Middle third of potentiometer's range (341-681)
{
potVal1 = ( (potVal1-341) * 3) / 4; // Normalize to 0-255
redVal = 0; // Red off
grnVal = 255 - potVal1; // Green from full to off
delay(potVal2); // Stop the program for some time
bluVal = potVal1; // Blue from off to full
delay(potVal2); // Stop the program for some time
}
else // Upper third of potentiometer"s range (682-1023)
{
potVal1 = ( (potVal1-683) * 3) / 4; // Normalize to 0-255
redVal = potVal1; // Red from off to full
delay(potVal2); // Stop the program for some time
grnVal = 0; // Green off
bluVal = 255 - potVal1; // Blue from full to off
delay(potVal2); // Stop the program for some time
}
analogWrite(redPin, redVal); // Write values to LED pins
analogWrite(grnPin, grnVal);
analogWrite(bluPin, bluVal);
}
Photos
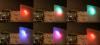
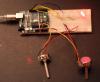
Comments
nice job! I like the photo
nice job! I like the photo series of the cross fading! Maybe we should have encouraged video submissions of everything :)