Components
Red, green, and blue LEDs
Wires x 4
220 ohm resistors x 3
Arduino board
Bread board
USB cable
Result in the in-lab exercises
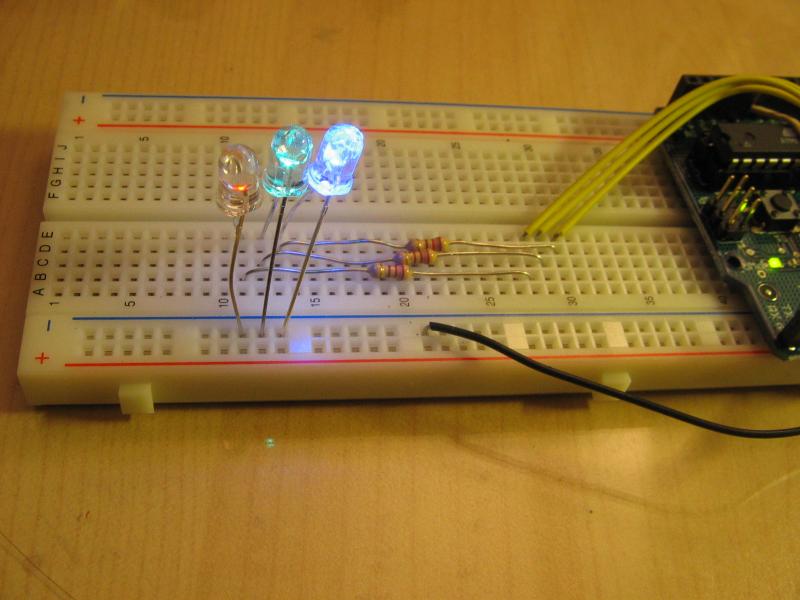
HOMEWORK 2)
I used the caps of my sun cream to be the diffuser.
HOMEWORK 3)
In this part, i use three variables redCount, greenCount, and blueCount to count how many repetitions of each r, g, b are. Then, i set the brightness based on the calcucated percentage.
Code
/*
* Serial RGB LED
* ---------------
* Serial commands control the brightness of R,G,B LEDs
* Command structure is "<colorCode>*", where "colorCode" is
* one of "r","g", or "b".
* For example, pressing ‘r’ 5 times will set the brightness to 50% (or brightness = 127)
* and pressing ‘r’ 10 times will set it to 100% (or brightness = 255).
*
*
*
*/
//include support for manipulating strings.
//for a useful string comparison function, see the bottom of this file... stringsEqual()
#include <stdio.h>
#include <string.h>
char serInString[100]; // array that will hold the different bytes of the string. 100=100characters;
// -> you must state how long the array will be else it won't work properly
char colorCode;
int colorVal;
int redPin = 9; // Red LED, connected to digital pin 9
int greenPin = 10; // Green LED, connected to digital pin 10
int bluePin = 11; // Blue LED, connected to digital pin 11
int redValue = 127;
int greenValue = 127;
int blueValue = 127;
void setup() {
pinMode(redPin, OUTPUT); // sets the pins as output
pinMode(greenPin, OUTPUT);
pinMode(bluePin, OUTPUT);
Serial.begin(9600);
analogWrite(redPin, redValue); // set them all to mid brightness
analogWrite(greenPin, greenValue); // set them all to mid brightness
analogWrite(bluePin, blueValue); // set them all to mid brightness
Serial.println("enter color command (e.g. 'r43 or rrrrrrrrbbbb') :");
}
void loop () {
//read the serial port and create a string out of what you read
readSerialString(serInString, 100);
//UNCOMMENT ONE OF THE FOLLOWING COMMANDS, OR NOTHING WILL HAPPEN WHEN YOU
//RUN THE PROGRAM...
myProcessRepeatKeyCommands(serInString, 100);
//Erase anything left in the serial string, preparing it for the
//next loop
resetSerialString(serInString, 100);
delay(100); // wait a bit, for serial data
}
void resetSerialString (char *strArray, int length) {
for (int i = 0; i < length; i++) {
strArray[i] = '\0';
}
}
//read a string from the serial and store it in an array
//you must supply the array variable
void readSerialString (char *strArray, int maxLength) {
int i = 0;
if(!Serial.available()) {
return;
}
while (Serial.available() && i < maxLength) {
strArray[i] = Serial.read();
i++;
}
}
void myProcessRepeatKeyCommands(char *strArray, int maxLength) {
int i = 0;
int redCount = 0, greenCount = 0, blueCount = 0;
//loop through the string (strArray)
//i = the current position in the string
//Stop when either (a) i reaches the end of the string or
// (b) there is an empty character '\0' in the string
while (i < maxLength && strArray[i] != '\0') {
//Read in the character at position i in the string
colorCode = serInString[i];
//If the character is r (red)...
if (colorCode == 'r') {
redCount ++;
//If the character is g (green)...
} else if (colorCode == 'g') {
greenCount ++;
//If the character is b (blue)...
} else if (colorCode == 'b') {
blueCount ++;
}
//Move on to the next character in the string
//From here, the code continues executing from the "while" line above...
i++;
}
if (i > 0) {
redValue = 255 * redCount / 10;
analogWrite(redPin, redValue);
Serial.print("setting color r to ");
Serial.println(redValue);
greenValue = 255 * greenCount / 10;
analogWrite(greenPin, greenValue);
Serial.print("setting color g to ");
Serial.println(greenValue);
blueValue = 255 * blueCount / 10;
analogWrite(bluePin, blueValue);
Serial.print("setting color b to ");
Serial.println(blueValue);
}
}
HOMEWORK 4)
Code
/*
* Serial RGB LED
* ---------------
* Serial commands control the brightness of R,G,B LEDs
* Command structure is "<colorCode>*", where "colorCode" is
* one of "r","g", or "b".
* For example, pressing ‘r’ 5 times will set the brightness to 50% (or brightness = 127)
* and pressing ‘r’ 10 times will set it to 100% (or brightness = 255).
*
*
*
*/
//include support for manipulating strings.
//for a useful string comparison function, see the bottom of this file... stringsEqual()
#include <stdio.h>
#include <string.h>
char serInString[100]; // array that will hold the different bytes of the string. 100=100characters;
// -> you must state how long the array will be else it won't work properly
char colorCode;
int colorVal;
int redPin = 9; // Red LED, connected to digital pin 9
int greenPin = 10; // Green LED, connected to digital pin 10
int bluePin = 11; // Blue LED, connected to digital pin 11
int redValue = 127;
int greenValue = 127;
int blueValue = 127;
int redCount = 5, greenCount = 5, blueCount = 5;
void setup() {
pinMode(redPin, OUTPUT); // sets the pins as output
pinMode(greenPin, OUTPUT);
pinMode(bluePin, OUTPUT);
Serial.begin(9600);
analogWrite(redPin, redValue); // set them all to mid brightness
analogWrite(greenPin, greenValue); // set them all to mid brightness
analogWrite(bluePin, blueValue); // set them all to mid brightness
Serial.println("enter color command (e.g. 'r43 or rrrrrrrrbbbb') :");
}
void loop () {
char c = Serial.read();
switch(c) {
case 'r':
redCount ++;
break;
case 'g':
greenCount ++;
break;
case 'b':
blueCount ++;
break;
default:
return;
}
redValue = 255 * (redCount % 10)/ 10;
greenValue = 255 * (greenCount % 10)/ 10;
blueValue = 255 * (blueCount % 10)/ 10;
analogWrite(redPin, redValue);
analogWrite(greenPin, greenValue);
analogWrite(bluePin, blueValue);
Serial.print("setting color (red, green, blue) to (");
Serial.print(redValue);
Serial.print(", ");
Serial.print(greenValue);
Serial.print(", ");
Serial.print(blueValue);
Serial.println(")");
}