Freeze!
Description
Use the Arduino to control a light emitting diode and make it blink.
Components Used
Light Emitting Diode (LED)--blue, red
Resistors
Piezo
FSR
Arduino Code
/* I said "Freeze!"
* I added a FSR into Paddington so that when he raises his arm to
* in hot pursuit of a thief, it will emit a whistle (like the
British bobbies */
int speakerPin = 7;
int ledPin1 = 13; // blue LED
int ledPin2 = 12; // red LED
int fsrPin = 0;
int fsrVal=0; //store value read from FSR
int tone = 956; //The "whistle" is in C
void setup()
{
pinMode(ledPin1, OUTPUT); // sets the digital pin as output for blue LED
pinMode(ledPin2, OUTPUT); // sets the digital pin as output for blue LED
pinMode(speakerPin, OUTPUT);
Serial.begin(9600);
}
void loop()
{
//load the value from the FSR
fsrVal = analogRead(fsrPin); // read the value from the FSR; 0 = arm up; > 0 = arm down
Serial.print("fsrVal "); // debug--what's the FSR reading?
Serial.print(fsrVal);
if (fsrVal > 0)
{
// if Paddington's arm is down, he's not in pursuit
digitalWrite(ledPin1, HIGH); // sets the blue LED on
digitalWrite(ledPin2,LOW); //sets the red LED off
delay(1000); // waits for a second
}
else
{
// if Paddington's in hot pursuit and waving his arm menacingly
// have the LED blink violently as if he's trying to pull-over a car
inPursuit();
}
}
//beep once and blink the LED
void inPursuit()
{
digitalWrite(ledPin1, LOW); // sets the blue LED on
digitalWrite(ledPin2, HIGH); //sets the red LED off
delay(100); // make the red light blink rapidly
for( int i=0; i<50; i++ )
{
digitalWrite(speakerPin, HIGH);
delayMicroseconds(tone);
digitalWrite(speakerPin, LOW);
delayMicroseconds(tone);
}
digitalWrite(ledPin2, LOW); //sets the red LED off
}
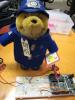
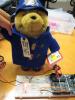
Paddington on Patrol (blue LED on)
Freeze! (Paddington in hot pursuit of fleeing suspect--red LED on and beep)